PyTorch?
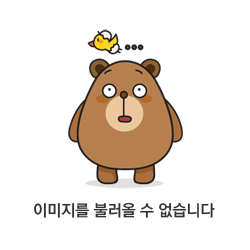
텐서플로우와 함께 대표적인 딥러닝 프레임워크이며 근래 유저의 사용량이 텐서플로우를 넘어서고 있음.
Define by Run
실행을 하며 그래프를 생성하는 방식으로 텐서플로우와 가장 비교되는 파이토치의 생성 방식. 이 때문에 교육용과 연구용 모두에서 파이토치를 사용하는 사람의 비중이 늘어나고 있음.
numpy + AutoGrad
numpy와 같은 구조를 가지는 Tensor 객체로 다차원 Array를 표현함. (numpy의 ndarray와 동일)
import numpy as np
n_array = np.arange(10).reshape(2,5)
print(n_array)
print("ndim :", n_array.ndim, "shape :", n_array.shape)
# [[0 1 2 3 4]
# [5 6 7 8 9]]
# ndim : 2 shape : (2, 5)
import torch
t_array = torch.FloatTensor(n_array)
print(t_array)
print("ndim :", t_array.ndim, "shape :", t_array.shape)
###
tensor([[0., 1., 2., 3., 4.],
[5., 6., 7., 8., 9.]])
ndim : 2 shape : torch.Size([2, 5])
###
두 결과는 같은 기능을 한다.
Array to Tensor
data = [[3, 5],[10, 5]]
x_data = torch.tensor(data)
x_data
###
tensor([[ 3, 5],
[10, 5]])
###
텐서로의 간단한 변경
nd_array_ex = np.array(data)
tensor_array = torch.from_numpy(nd_array_ex)
tensor_array
###
tensor([[ 3, 5],
[10, 5]])
###
ndarray 역시 변경 가능하다.
Tensor handling
- view : reshape와 동일하게 tensor의 shape를 변환하는 기능
- squeeze : 차원의 개수가 1인 차원을 삭제 (압축하는 기능)
- unsqueeze : 차원의 개수가 1인 차원을 추가하는 기능
tensor_ex = torch.rand(size=(2, 3, 2))
tensor_ex
###
tensor([[[0.0959, 0.7055],
[0.3562, 0.6360],
[0.5473, 0.8004]],
[[0.5079, 0.5433],
[0.1673, 0.2446],
[0.6965, 0.4139]]])
###
의 예제 tensor 객체를 생성
tensor_ex.view([-1, 6])
tensor_ex.reshape([-1,6])
# 모두 같은 결과를 출력
tensor([[0.0959, 0.7055, 0.3562, 0.6360, 0.5473, 0.8004],
[0.5079, 0.5433, 0.1673, 0.2446, 0.6965, 0.4139]])
view와 reshape의 차이?
contiquity 보장의 차이 (인접성)
a = torch.zeros(3, 2)
b = a.view(2, 3)
a.fill_(1)
를 통해 a라는 0으로 이루어진 3행 2열 텐서를 만들고 view를 통해 2행 3열로 바꾼 b라는 텐서를 생성.
a.fill을 통해 1로 값을 가득 채우는 경우
a
>>> tensor([[1., 1.],
[1., 1.],
[1., 1.]])
b
>>> tensor([[1., 1., 1.],
[1., 1., 1.]])
a와 b 모두 a.fill_(1)의 영향을 받는다.
반면
a = torch.zeros(3, 2)
b = a.t().reshape(6)
a.fill_(1)
a
>>> tensor([[1., 1.],
[1., 1.],
[1., 1.]])
b
>>> tensor([0., 0., 0., 0., 0., 0.])
reshape의 경우 0행 6열의 평활화를 진행하는 경우 b에 a.fill_(1)의 영향을 받지 않아 0으로 이루어진 텐서가 생성된다.
squeeze & unsqueeze
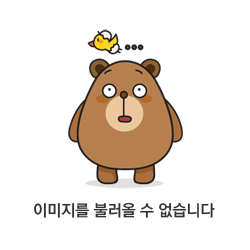
해당 그림처럼 unsqueeze를 사용할 때
unsqueeze(인자) 의 인자에 0, 1, 2 등의 값을 넣을 수 있다. 이는 판다스의 axis = 0, 1, 2와 같은 개념으로
unsqueeze를 할 축을 설정하는 기능을 한다.
행렬곱의 연산
일반적인 행렬곱 연산인 dot을 쓰지 않고 mm이라는 함수를 사용
n2 = np.arange(10).reshape(5,2)
t2 = torch.FloatTensor(n2)
t1.mm(t2)
>>> tensor([[ 60., 70.],
[160., 195.]])
t1.dot(t2)
>>> ---------------------------------------------------------------------------
RuntimeError Traceback (most recent call last)
~\AppData\Local\Temp/ipykernel_7364/2372691299.py in <module>
----> 1 t1.dot(t2)
RuntimeError: 1D tensors expected, but got 2D and 2D tensors
t1.matmul(t2)
>>> tensor([[ 60., 70.],
[160., 195.]])
mm과 matmul은 같은 결과를 출력하며, dot은 차원이 맞지 않는 오류가 남
mm과 matmul은 broadcasting의 지원 차이가 있음
broadcasting이란 자동으로 연산할 때 두 행렬간의 차원을 맞춰주는 기능.
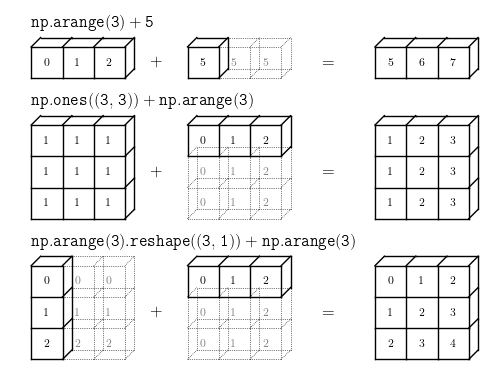
AutoGrad
PyTorch의 핵심기능인 자동 미분의 지원은 backward함수를 사용해 구할 수 있음
w = torch.tensor(2.0, requires_grad=True)
y = w**2
z = 10*y + 50
z.backward()
w.grad
>>> tensor(40.)
requires_grad = True 인자를 통해 자동 미분을 구현할 수 있음.
위 코드는
의 예시임
'네이버 부스트캠프 학습 정리 > 2주차' 카테고리의 다른 글
[PyTorch] PyTorch 알쓸신잡 (0) | 2023.03.19 |
---|---|
[PyTorch] PyTorch의 데이터 (0) | 2023.03.19 |
[PyTorch] parameter & buffer (0) | 2023.03.19 |
[PyTorch] nn.Module (0) | 2023.03.17 |